core.h File Reference
File containing the core that generates the solutions. More...
#include <stdio.h>
#include <math.h>
#include "../types/model.h"
#include "../types/examples.h"
#include "../types/solution.h"
#include "../types/object-index.h"
#include "output.h"
Include dependency graph for core.h:
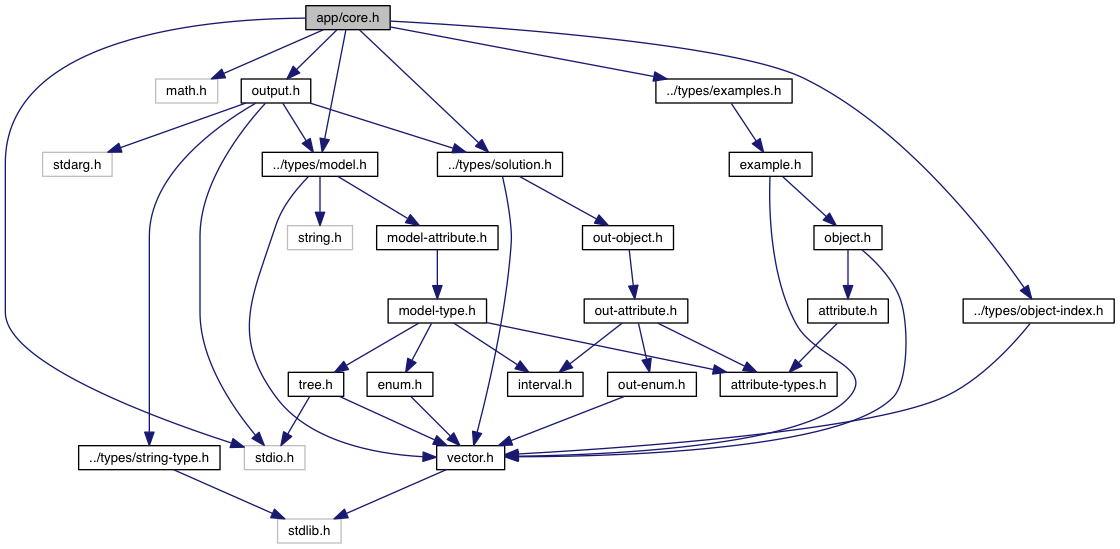
Go to the source code of this file.
Functions | |
int | nbCombi (Examples *exp, int expIndice) |
Computes the number of combinations possible for our examples from an example. More... | |
OutObject * | initOutObjectWithObject (Model *mdl, Object *o) |
Generate a filled OutObject based on an object values. More... | |
Solution * | initAllCombi (Model *mdl, Examples *exp) |
Init all combinations with last example objects. More... | |
void | combiOutObjectObject (Model *mdl, OutObject *oo, Object *o) |
Combine an OutObject and an Object into an OutObject. More... | |
Solution * | genAllCombi (Model *mdl, Examples *exp) |
Generate all the combinations for our examples. More... | |
void | genAllRelations (Solution *s, Examples *e, Model *m) |
Find all the common relation between the objects. More... | |
int | getIndex (Examples *exp, ObjectIndex *oi) |
Get the index in the array where the combinations of objects are stored \(n\): number of example-1 \(X_i\): index of the object in the example i \(O_j\): number of objects in the example j And our continuous bijection (homomorphism) formula: \((\sum\limits_{i=0}^{n-1} (X_i*\prod\limits_{j=i+1}^{n} O_j))+X_n\). More... | |
int | isOutObjectIncludeInAnother (Model *mdl, OutObject *oo1, OutObject *oo2) |
Is oo1 include in oo2? More... | |
void | genGeneralization (Model *mdl, Solution *s) |
generalization of our solution(s) More... | |
void | genCounterExamples (Model *m, Examples *e, Solution *s) |
Add counter-examples to the solutions. More... | |
int | isObjectInOutObject (Model *m, OutObject *oo, Object *o) |
Check whether an OutObject matches an object or not. More... | |
Detailed Description
File containing the core that generates the solutions.
Function Documentation
Find all the common relation between the objects.
- Parameters
-
s The solution generated by the genAllCombi function e The examples to search the relations into m The model to use the relations
Add counter-examples to the solutions.
- Parameters
-
m Pointer to the Model e Pointer to the examples s Pointer to the solution
Here is the call graph for this function:

int getIndex | ( | Examples * | exp, |
ObjectIndex * | oi | ||
) |
Get the index in the array where the combinations of objects are stored
\(n\): number of example-1
\(X_i\): index of the object in the example i
\(O_j\): number of objects in the example j
And our continuous bijection (homomorphism) formula:
\((\sum\limits_{i=0}^{n-1} (X_i*\prod\limits_{j=i+1}^{n} O_j))+X_n\).
- Parameters
-
exp Pointer to the examples oi Pointer to the objects's indexes
- Returns
- An integer
Here is the caller graph for this function:

int nbCombi | ( | Examples * | exp, |
int | expIndice | ||
) |
Computes the number of combinations possible for our examples from an example.
- Parameters
-
exp Pointer to our array of exemple expIndice The example number
- Returns
- The number of combinations starting at expIndice example
Computes the number of combinations possible for our examples from an example.
Here is the caller graph for this function:
