Contains the output functions, helpers and functions to create and display human readable strings. More...
#include <stdio.h>
#include <stdarg.h>
#include "../types/model.h"
#include "../types/solution.h"
#include "../types/string-type.h"
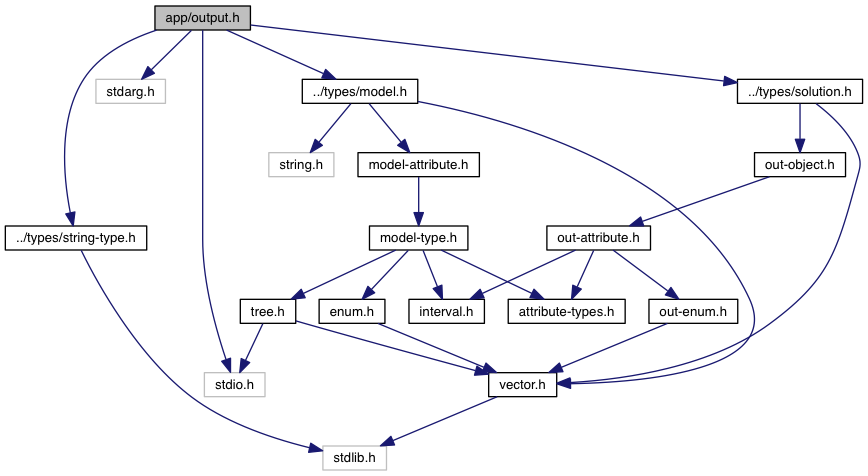
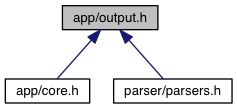
Go to the source code of this file.
Macros | |
#define | LERROR 8 |
Flag for the output function. Represents an error. | |
output importance | |
Flags for the output function. Represents levels of importance | |
#define | L0 0 |
#define | L1 1 |
#define | L2 2 |
#define | L3 3 |
#define | L4 4 |
#define | L5 5 |
#define | L6 6 |
#define | L7 7 |
Functions | |
void | genOutput (Solution *sol, Model *mdl, int recur) |
Returns a string that contains the representation of the object in a readable presentation. More... | |
void | genObjectOutput (OutObject *oo, Model *m, int recur) |
Print an OutObject. More... | |
char * | cPrint (const char *fmt,...) |
Returns a string that contains the formatted output by concatening all the arguments. More... | |
void | setOutputImportance (unsigned int level) |
Sets the max level of the messages to output (0 : only critical messages, the higher the value, the less importance the messages) More... | |
unsigned int | getOutputImportance () |
Gets the max level of the messages to output (0 : only critical messages, the higher the value, the less importance the messages) | |
void | output (unsigned int level, const char *fmt,...) |
Print the message in the standard output only if its importance is high enough to be printed. More... | |
unsigned int | extractVerbosityFromArg (const char *verbosity) |
Extract the verbosity value from the "-v[v..]" formated string. More... | |
void | enableColors (unsigned int enable) |
Enables or not the colors in the output. More... | |
void | printNChar (unsigned int level, char c, unsigned int n) |
Print a fixed number of time a character. More... | |
Detailed Description
Contains the output functions, helpers and functions to create and display human readable strings.
Function Documentation
char* cPrint | ( | const char * | fmt, |
... | |||
) |
Returns a string that contains the formatted output by concatening all the arguments.
- Parameters
-
fmt The format string (same used by the printf family) ... The list of arguments to include in the output string
- Returns
- A newly allocated string that contains the arguments given to the function formated
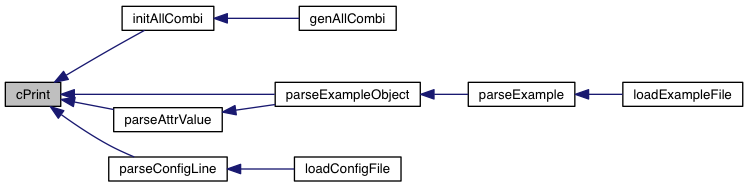
void enableColors | ( | unsigned int | enable | ) |
Enables or not the colors in the output.
- Parameters
-
enable Boolean. If enable = 0, the colors are disabled, colors are activated for any other values
unsigned int extractVerbosityFromArg | ( | const char * | verbosity | ) |
Extract the verbosity value from the "-v[v..]" formated string.
- Parameters
-
verbosity The string to extract the verbosity from
- Returns
- The level extracted
Returns a string that contains the representation of the object in a readable presentation.
- Parameters
-
sol Pointer to the object grouping the common traits of the other objects int the examples mdl Pointer to the model object containing structure of the model recur Boolean to presize wheter relations must be printed recursively or just their name (0 : no recursion, 1: recursion)
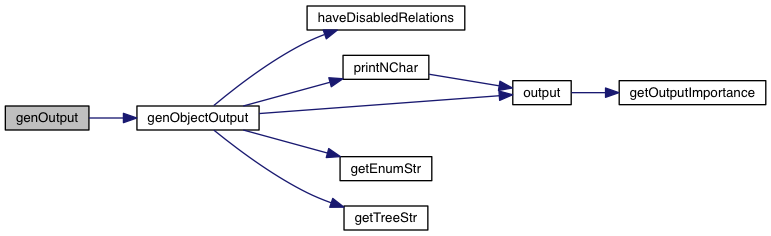
void output | ( | unsigned int | level, |
const char * | fmt, | ||
... | |||
) |
Print the message in the standard output only if its importance is high enough to be printed.
- Parameters
-
level The importance level of the message (flags, can use L[0-7] and add the flag LERROR if you want to write in the error stream LERROR alone is aquivalent to L0 | LERROR fmt The message to be printed ... The arguments needed by the fmt argument

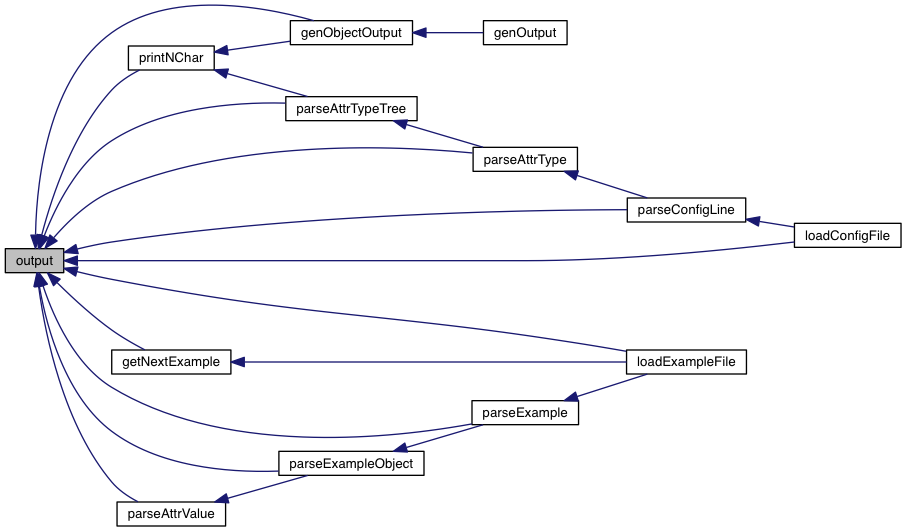
void printNChar | ( | unsigned int | level, |
char | c, | ||
unsigned int | n | ||
) |
Print a fixed number of time a character.
- Parameters
-
level The importance level of the message (flags, can use L[0-7] and add the flag LERROR if you want to write in the error stream LERROR alone is aquivalent to L0 | LERROR c The character to print n The number of time to print the character


void setOutputImportance | ( | unsigned int | level | ) |
Sets the max level of the messages to output (0 : only critical messages, the higher the value, the less importance the messages)
- Parameters
-
level The level to set