Contains the example and model file parser. More...
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <limits.h>
#include "../types/examples.h"
#include "../types/model.h"
#include "../types/string-type.h"
#include "../app/output.h"
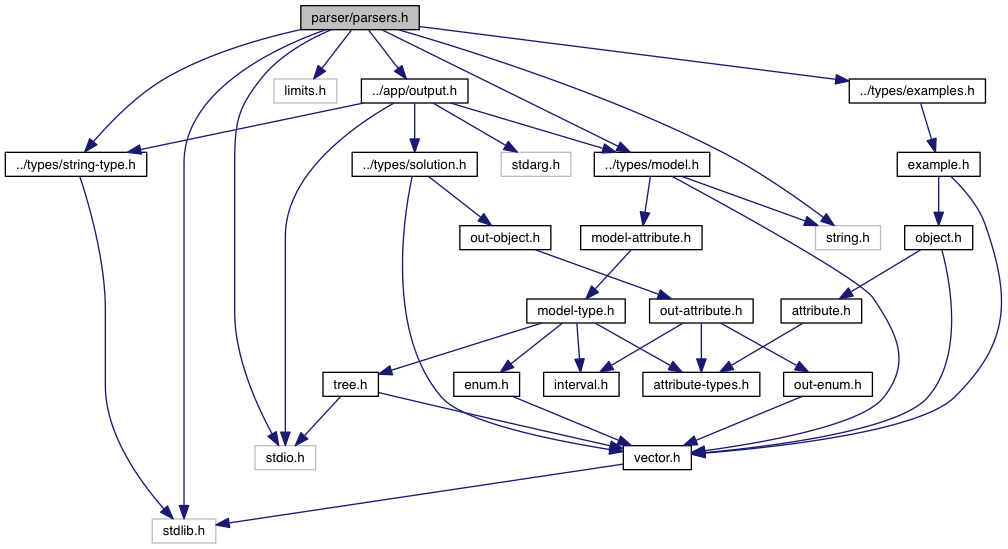
Go to the source code of this file.
Data Structures | |
struct | StringVector |
Stores an array of C string. More... | |
Macros | |
#define | PARSED_EXAMPLE 1 |
Value representing an example in the example file. | |
#define | PARSED_COUNTEREXAMPLE 2 |
Value representing a counter-example in the example file. | |
Functions | |
char * | getIncludeFile (char const *pathname, size_t *pos) |
Get the pathname to the config file included at the begening of an example file. More... | |
Examples * | loadExampleFile (char const *pathname, Model *model, size_t startPos) |
Loads the example file given and generate the Example object that represents its content. More... | |
unsigned int | getNextExample (FILE *f) |
Get the type of the next example (example or counter-example). Stop reading at the end of the example name, on the last character. More... | |
int | parseExample (FILE *fp, char **error, Example *ex, Model *m) |
Parse an example or a counterexample. More... | |
int | parseExampleObject (FILE *fp, char **error, Object *o, Model *m, struct StringVector *seenObjects) |
Parse an object (only its properties. The name must already be known) More... | |
int | getAttributePosition (const char *attr, Model *m) |
Returns the position at which can be found an attribute (by name) More... | |
int | getRelationPosition (const char *rel, Model *m) |
Returns the position at which can be found a relation (by name) More... | |
void | parseAttrValue (FILE *fp, char **error, Model *m, attrType type, Attribute *attr, unsigned int position, struct StringVector *seenObjects) |
Parse the attribute's value and populate the Attribute object accordingly. More... | |
Model * | loadConfigFile (char const *pathname) |
Loads the config file given anf the generate the Model object that represents its content. More... | |
int | parseConfigLine (FILE *fp, char **error, Model *out) |
Tries to parse the line as a config file attribute definition. If the line is empty, continues to read until it finds a line. More... | |
char * | parseAttrName (FILE *fp, char **error) |
Tries to parse the attribute name at the current position in the file (spaces & tabs are ommited) More... | |
ModelType * | parseAttrType (FILE *fp, char **error) |
Tries to parse the attribute's value definition at the current position in the file (may read more than one line in case of trees) More... | |
Interval * | parseAttrTypeInterval (FILE *fp, char **error) |
Tries to parse an interval. More... | |
Enum * | parseAttrTypeEnum (FILE *fp, char **error) |
Tries to parse an enumeration. More... | |
Tree * | parseAttrTypeTree (FILE *fp, char **error, int *index, int indent) |
Tries to parse a tree. More... | |
int | isValidAttrChar (char c, unsigned int first) |
Check whether the character is allowed in an attribute name or not. More... | |
void | readFileSpaces (FILE *fp, char const *set) |
Reads a file from the current position and reads while characters are in the set. Stops on the last one. More... | |
void | readTil (FILE *fp, char const *set) |
Reads a file from the current position and reads until it finds a character in the set. Stops on the last character not in the set. More... | |
Detailed Description
Contains the example and model file parser.
Function Documentation
int getAttributePosition | ( | const char * | attr, |
Model * | m | ||
) |
Returns the position at which can be found an attribute (by name)
- Parameters
-
attr the attribute to search for m The model in which to find the order
- Returns
- the index of the attribute (or -1 in case the attributes is not in the model)

char* getIncludeFile | ( | char const * | pathname, |
size_t * | pos | ||
) |
Get the pathname to the config file included at the begening of an example file.
- Parameters
-
pathname The path to the example file pos Will contain the position of the character after the last character of the include (basically, a " ", "\t" or "\n")
- Returns
- If the file to include is found, the file name. NULL otherwise
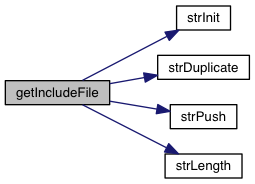
unsigned int getNextExample | ( | FILE * | f | ) |
Get the type of the next example (example or counter-example). Stop reading at the end of the example name, on the last character.
- Parameters
-
f The file to be read
- Returns
- 0 in case of error, PARSED_EXAMPLE if the line is an example, PARSED_COUNTEREXAMPLE if the line is a counter-example


int getRelationPosition | ( | const char * | rel, |
Model * | m | ||
) |
Returns the position at which can be found a relation (by name)
- Parameters
-
rel the relation to search for m The model in which to find the order
- Returns
- the index of the attribute (or -1 in case the attributes is not in the model)

int isValidAttrChar | ( | char | c, |
unsigned int | first | ||
) |
Check whether the character is allowed in an attribute name or not.
- Parameters
-
c The character to Check first whether the character is the first to be read or not
- Returns
- Returns 1 if the character is valid. 0 otherwise
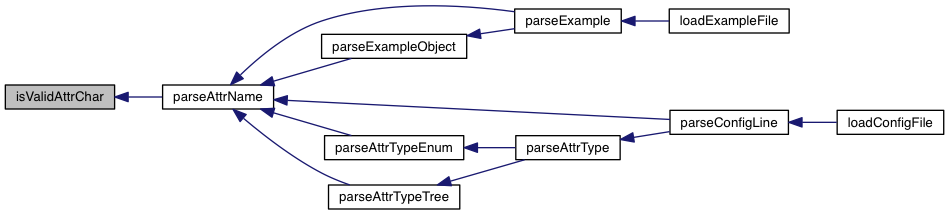
Model* loadConfigFile | ( | char const * | pathname | ) |
Loads the example file given and generate the Example object that represents its content.
- Parameters
-
pathname The path to the example file to be read model The Model object generated from the config file startPos The position at which to start parsing the file
- Returns
- A newly created Examples object
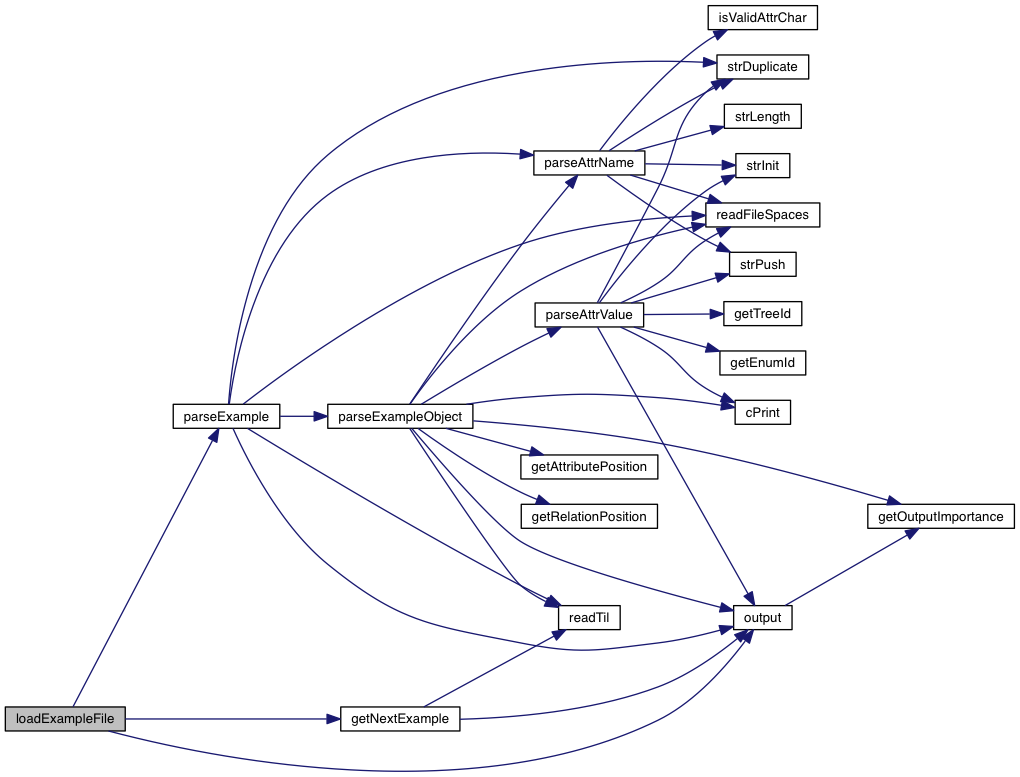
char* parseAttrName | ( | FILE * | fp, |
char ** | error | ||
) |
Tries to parse the attribute name at the current position in the file (spaces & tabs are ommited)
- Parameters
-
fp Ther file in which to read error In case of error, contains a description of the error. NULL if no error append. Must be an uninitialized variable or data loss may happen
- Returns
- Returns a new string that contains the attribute name or NULL in case of error
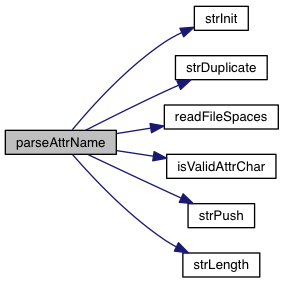
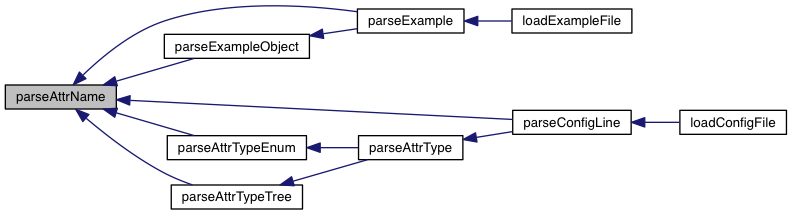
ModelType* parseAttrType | ( | FILE * | fp, |
char ** | error | ||
) |
Tries to parse the attribute's value definition at the current position in the file (may read more than one line in case of trees)
- Parameters
-
fp Ther file in which to read error In case of error, contains a description of the error. NULL if no error append. Must be an uninitialized variable or data loss may happen
- Returns
- Returns the ModelType built from the file definition or NULL in case of error
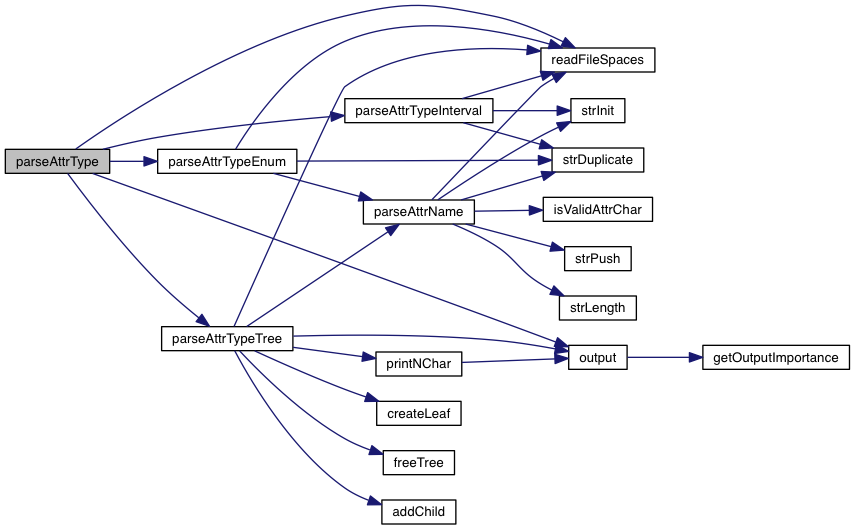

Enum* parseAttrTypeEnum | ( | FILE * | fp, |
char ** | error | ||
) |
Tries to parse an enumeration.
- Parameters
-
fp Ther file in which to read error In case of error, contains a description of the error. NULL if no error append. Must be an uninitialized variable or data loss may happen
- Returns
- The parsed value or NULL in case of error
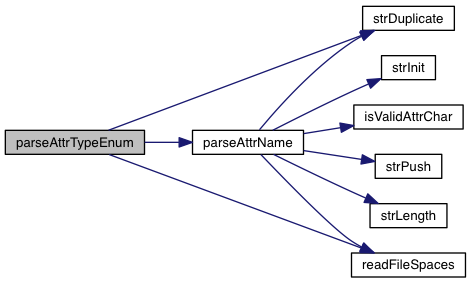

Interval* parseAttrTypeInterval | ( | FILE * | fp, |
char ** | error | ||
) |
Tries to parse an interval.
- Parameters
-
fp Ther file in which to read error In case of error, contains a description of the error. NULL if no error append. Must be an uninitialized variable or data loss may happen
- Returns
- The parsed value or NULL in case of error
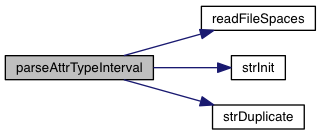

Tree* parseAttrTypeTree | ( | FILE * | fp, |
char ** | error, | ||
int * | index, | ||
int | indent | ||
) |
Tries to parse a tree.
- Parameters
-
fp Ther file in which to read error In case of error, contains a description of the error. NULL if no error append. Must be an uninitialized variable or data loss may happen index Pointer to the counter of nodes (id) indent Number of tab to print
- Returns
- The parsed value or NULL in case of error
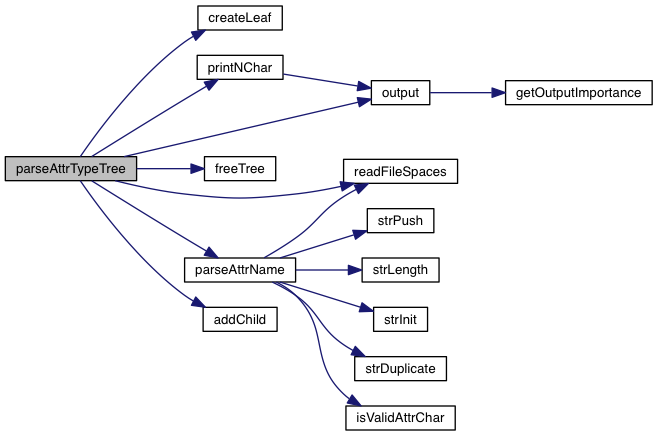

void parseAttrValue | ( | FILE * | fp, |
char ** | error, | ||
Model * | m, | ||
attrType | type, | ||
Attribute * | attr, | ||
unsigned int | position, | ||
struct StringVector * | seenObjects | ||
) |
Parse the attribute's value and populate the Attribute object accordingly.
- Parameters
-
fp The file in which to read error In case of error, contains a description of the error. NULL if no error happened. Must be an uninitialized variable or data loss may occur. m The model to use for the parsing type The expected type of the attribute attr A pointer to the attribute to populate position The position of the attribute in the model seenObjects The names of the objects that have already been seen
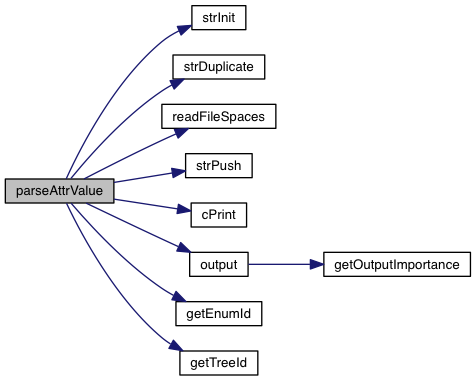

int parseConfigLine | ( | FILE * | fp, |
char ** | error, | ||
Model * | out | ||
) |
Tries to parse the line as a config file attribute definition. If the line is empty, continues to read until it finds a line.
- Parameters
-
fp The file in which to read error In case of error, contains a description of the error. NULL if no error append. Must be an uninitialized variable or data loss may happen out A pointer to the Model object to populate
- Returns
- A boolean. 1 for success. 0 for failure.
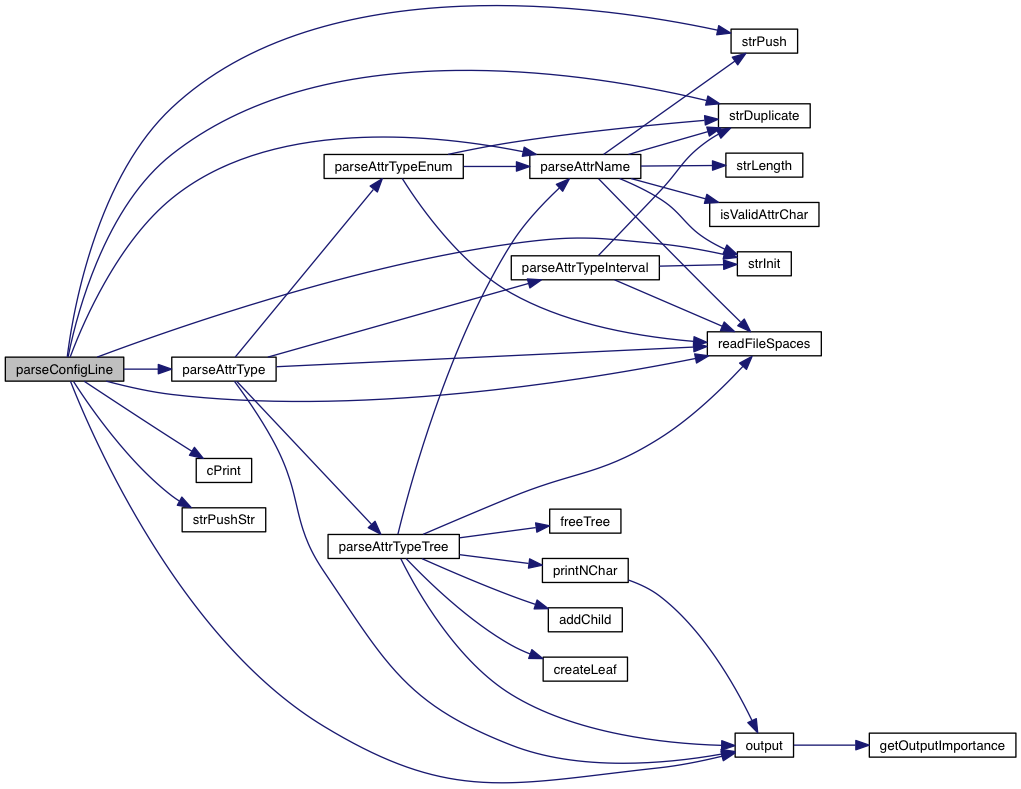

Parse an example or a counterexample.
- Parameters
-
fp The file in which to read error In case of error, contains a description of the error. NULL if no error happened. Must be an uninitialized variable or data loss may occur. ex A pointer to the example object to populate m The Model object generated from the config file
- Returns
- A boolean. 1 for success. 0 for failure.
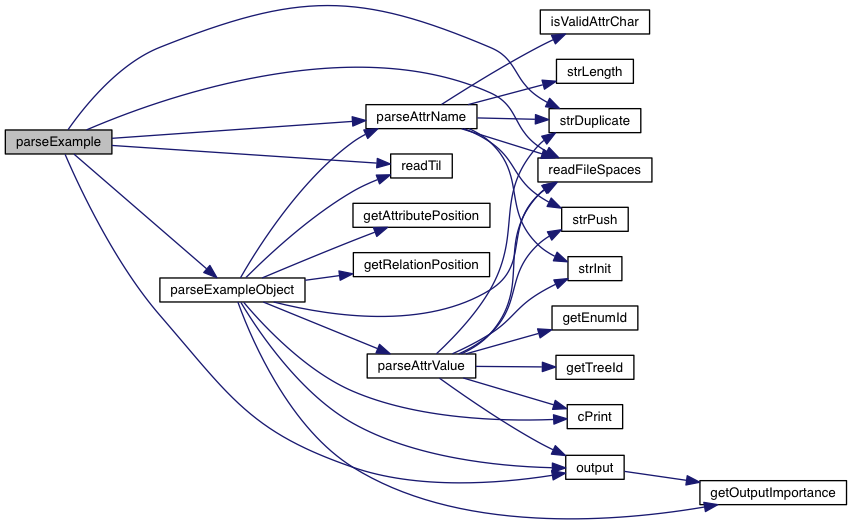

int parseExampleObject | ( | FILE * | fp, |
char ** | error, | ||
Object * | o, | ||
Model * | m, | ||
struct StringVector * | seenObjects | ||
) |
Parse an object (only its properties. The name must already be known)
- Parameters
-
fp The file in which to read error In case of error, contains a description of the error. NULL if no error happened. Must be an uninitialized variable or data loss may occur. o A pointer to the object Object to populate m The Model object generated from the config file seenObjects The names of the objects that have already been seen
- Returns
- A boolean. 1 for success. 0 for failure
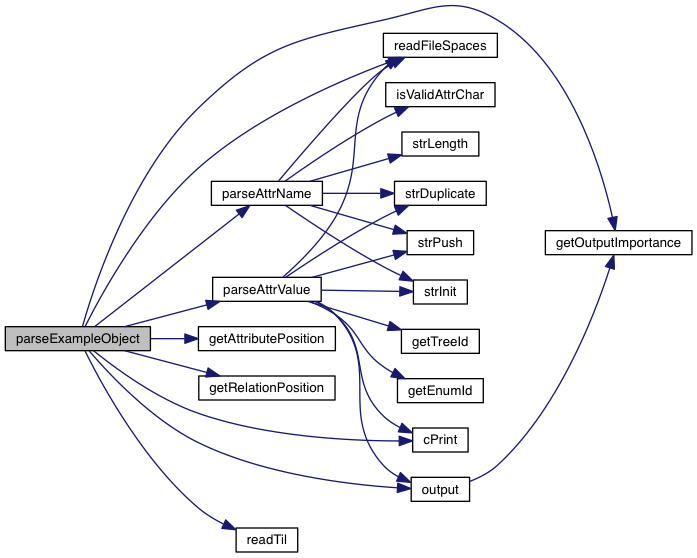

void readFileSpaces | ( | FILE * | fp, |
char const * | set | ||
) |
Reads a file from the current position and reads while characters are in the set. Stops on the last one.
- Parameters
-
fp The file to be read set A nul terminated array of char that contains the set of characters
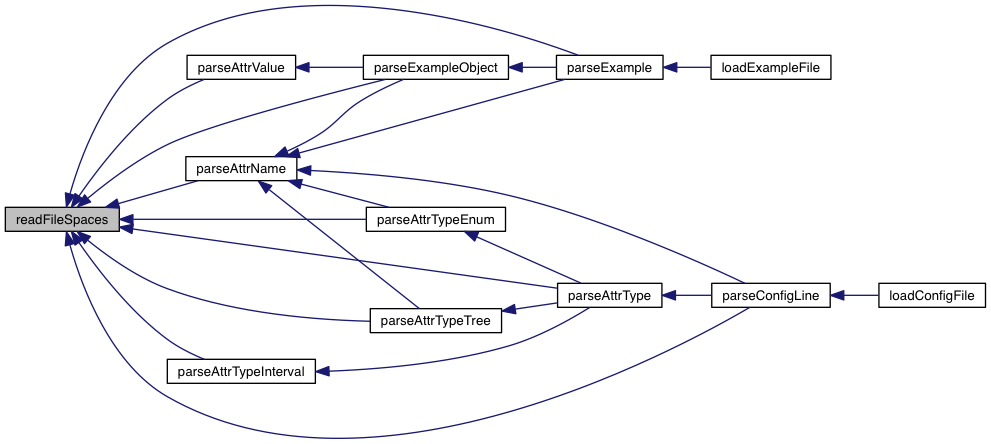
void readTil | ( | FILE * | fp, |
char const * | set | ||
) |
Reads a file from the current position and reads until it finds a character in the set. Stops on the last character not in the set.
- Parameters
-
fp The file to be read set A nul terminated array of char that contains the set of characters to reach
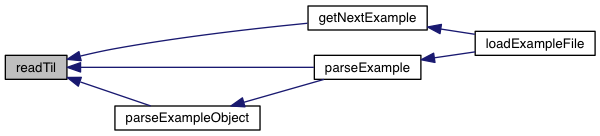