vector.h File Reference
Contains the definition of the vectors (dynamic & generic arrays) More...
#include <stdlib.h>
Include dependency graph for vector.h:
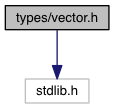
This graph shows which files directly or indirectly include this file:
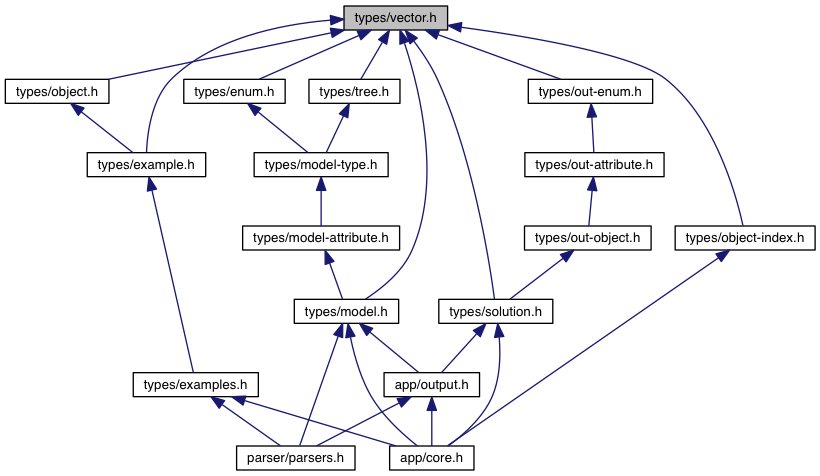
Go to the source code of this file.
Macros | |
#define | Vector(t) struct {int size, capacity; t *data; } |
Define a dynamic and generic array. More... | |
#define | vectInit(vect) ((vect).size = (vect).capacity = 0, (vect).data = 0) |
Init the vector. More... | |
#define | vectFree(vect) free((vect).data) |
Free the vector. More... | |
#define | vectAt(vect, index) ((vect).data[(index)]) |
Returns the element at a certain index of the array. More... | |
#define | vectSize(vect) ((vect).size) |
Return the size of the array. More... | |
#define | vectPush(type, vect, value) |
Append an element at the end of the vector. More... | |
#define | vectIndexOf(vect, value, out) |
Search for an element in the vector. Returns its index if found. More... | |
#define | vectRemoveLast(vect) |
Remove the last element in the vector. More... | |
#define | vectResize(type, vect, sizeToIncrease) |
Increase a vector of size. More... | |
#define | vectFill(type, vect, sizeToInit) |
Init the size of a vector to a prefined size. More... | |
Detailed Description
Contains the definition of the vectors (dynamic & generic arrays)
Macro Definition Documentation
#define vectAt | ( | vect, | |
index | |||
) | ((vect).data[(index)]) |
Returns the element at a certain index of the array.
- Parameters
-
vect The vector of which to access the element index The index of the element (between 0 and vector size - 1)
- Returns
- The element. You can use this as a left value.
#define vectFill | ( | type, | |
vect, | |||
sizeToInit | |||
) |
Value:
do { \
(vect).size = (vect).capacity = (sizeToInit); \
(vect).data = (type*)malloc(sizeof(type) * (sizeToInit)); \
} while(0) \
Init the size of a vector to a prefined size.
- Parameters
-
type The type of the element (must be the same type as the other elements of the array) vect The vector to init the size sizeToInit The size to init with
#define vectFree | ( | vect | ) | free((vect).data) |
Free the vector.
- Parameters
-
vect The vector to be freed
#define vectIndexOf | ( | vect, | |
value, | |||
out | |||
) |
Value:
do { \
out = -1; \
for(int _i = 0; _i < vectSize(vect); ++_i) { \
if(vectAt(vect, _i) == value) { \
out = _i; \
break; \
} \
} \
} while(0)
#define vectAt(vect, index)
Returns the element at a certain index of the array.
Definition: vector.h:40
Search for an element in the vector. Returns its index if found.
- Parameters
-
vect The vector to search in value The value to search for out An integer that will hold the return value (either the index if found ou -1 if the element is not int the vector)
#define vectInit | ( | vect | ) | ((vect).size = (vect).capacity = 0, (vect).data = 0) |
Init the vector.
- Parameters
-
vect The vector to be initialized
#define Vector | ( | t | ) | struct {int size, capacity; t *data; } |
Define a dynamic and generic array.
- Parameters
-
t The type of the items to store in the vector size The actual size of the vector, capacity The size allocated in the memory
#define vectPush | ( | type, | |
vect, | |||
value | |||
) |
Value:
do { \
if((vect).size == (vect).capacity) { \
(vect).capacity = ((vect).capacity ? (vect).capacity * 2 : 10); \
(vect).data = (type*)realloc((vect).data, sizeof(type) * (vect).capacity); \
} \
(vect).data[(vect).size++] = value; \
} while(0)
Append an element at the end of the vector.
- Parameters
-
type The type of the element (must be the same type as the other elements of the array) vect The vector at the end of which to add the element value The element to be appened
#define vectRemoveLast | ( | vect | ) |
Value:
do { \
if((vect).size > 0) { \
--(vect).size; \
} \
} while(0)
Remove the last element in the vector.
- Parameters
-
vect The vector to which remove the last element
#define vectResize | ( | type, | |
vect, | |||
sizeToIncrease | |||
) |
Value:
do { \
if(((sizeToIncrease)+(vect).size) < (vect).capacity) { \
(vect).size += sizeToIncrease; \
} else { \
(vect).data = (type*)realloc((vect).data, sizeof(type) * (sizeToIncrease)); \
(vect).size += (sizeToIncrease); \
(vect).capacity += (sizeToIncrease); \
} \
} while(0) \
Increase a vector of size.
- Parameters
-
type The type of the element (must be the same type as the other elements of the array) vect The vector to increase the size sizeToIncrease The size to increase
#define vectSize | ( | vect | ) | ((vect).size) |
Return the size of the array.
- Parameters
-
vect The array of which to get the size
- Returns
- The size of the array